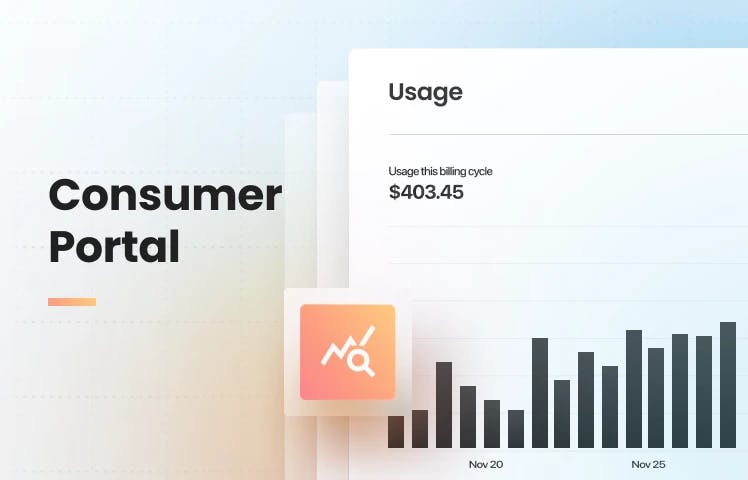
A tight feedback loop between customers interacting with your product and the usage reflected on their billing and usage dashboard is essential for controlling spending. The lack of instant usage feedback can lead to unexpected customer costs, overspending, and, eventually, churn. This is particularly critical in sectors like automation, AI, and APIs, where unforeseen software issues on the user's end can rapidly escalate usage and costs.
The OpenMeter Consumer Portal and Web SDK enable you to offer real-time usage dashboard for your customers, allowing them to track their consumption in real-time.
How can OpenMeter help?
With our Consumer Portal, you can build in-app user-facing dashboards where your customers can follow their consumption in real-time, backed by OpenMeter's robust metering. We provide the authentication layer and React hooks to fetch data from OpenMeter for specific customers so you can focus on building your product.
Getting Started
You can start using the Consumer Portal with React in three simple steps:
1. Create a subject-specific token
Use our SDKs to create a subject-specific token on your backend and return it to your frontend app.
2. Install the React.js Provider
Use our Web and React SDK to initialize the OpenMeter Provider with the generated token in your frontend app.
3. Query Usage With React Hooks
Use our React hooks to query OpenMeter.
Check out our Consumer Portal Guide and our Next.js dashboard example.
Get started today
We're excited to see what you'll build with OpenMeter!
Visit the
Consumer Portal to get started.